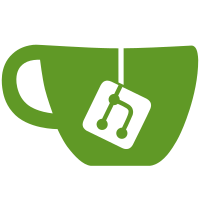
10 changed files with 228 additions and 182 deletions
@ -0,0 +1,44 @@
@@ -0,0 +1,44 @@
|
||||
let thread_id = null; |
||||
let exports = null; |
||||
|
||||
async function init(tid, memory, heap_base) { |
||||
thread_id = tid; |
||||
|
||||
const result = await WebAssembly.instantiateStreaming(fetch('wasm/lod.wasm'), { |
||||
env: { 'memory': memory } |
||||
}); |
||||
|
||||
exports = result.instance.exports; |
||||
exports.set_sp(heap_base - thread_id * 16 * 4096); // 64K stack
|
||||
|
||||
postMessage({ 'type': 'init_done' }); |
||||
} |
||||
|
||||
function work(indices_base, indices_count, zoom, offsets) { |
||||
exports.do_lod( |
||||
indices_base, indices_count, zoom, |
||||
offsets['coords_from'], |
||||
offsets['line_threshold'], |
||||
offsets['xs'], |
||||
offsets['ys'], |
||||
offsets['pressures'], |
||||
offsets['result_buffers'] + thread_id * 4, |
||||
offsets['result_counts'] + thread_id * 4, |
||||
); |
||||
|
||||
postMessage({ 'type': 'lod_done' }); |
||||
} |
||||
|
||||
onmessage = (e) => { |
||||
const d = e.data; |
||||
|
||||
if (d.type === 'init') { |
||||
init(d.thread_id, d.memory, d.heap_base); |
||||
} else if (d.type === 'lod') { |
||||
work(d.indices_base, d.indices_count, d.zoom, d.offsets); |
||||
} else { |
||||
console.error('unexpected worker command:', d.type); |
||||
} |
||||
} |
||||
|
||||
|
@ -1 +1 @@
@@ -1 +1 @@
|
||||
clang -Oz --target=wasm32 -nostdlib -msimd128 -mbulk-memory -matomics -Wl,--no-entry,--import-memory,--shared-memory,--max-memory=$((1024 * 1024 * 1024)) -z stack-size=$((1024 * 1024)) multi.c -o multi.wasm |
||||
clang -Oz --target=wasm32 -nostdlib -msimd128 -mbulk-memory -matomics -Wl,--no-entry,--import-memory,--shared-memory,--export-all,--max-memory=$((1024 * 1024 * 1024)) -z stack-size=$((1024 * 1024)) lod.c -o lod.wasm |
||||
|
Binary file not shown.
@ -1,37 +0,0 @@
@@ -1,37 +0,0 @@
|
||||
#include <wasm_simd128.h> |
||||
|
||||
extern char __heap_base; |
||||
|
||||
static int allocated; |
||||
|
||||
void |
||||
set_sp(char *sp) |
||||
{ |
||||
__asm__ __volatile__( |
||||
".globaltype __stack_pointer, i32\n" |
||||
"local.get %0\n" |
||||
"global.set __stack_pointer\n" |
||||
: : "r"(sp) |
||||
); |
||||
} |
||||
|
||||
void * |
||||
alloc(int size) |
||||
{ |
||||
void *result = &__heap_base + allocated; |
||||
allocated += size; |
||||
return(result); |
||||
} |
||||
|
||||
static void |
||||
impl(int *buffer, int index, int number) |
||||
{ |
||||
buffer[index] = number; |
||||
} |
||||
|
||||
void |
||||
write_a_number(int *buffer, int index, int number) |
||||
{ |
||||
int n = number * 2; |
||||
impl(buffer, index, n); |
||||
} |
Binary file not shown.
@ -1,40 +0,0 @@
@@ -1,40 +0,0 @@
|
||||
let thread_id = null; |
||||
let buf_offset = null; |
||||
let exports = null; |
||||
let flags = null; |
||||
|
||||
function done() { |
||||
postMessage('done'); |
||||
} |
||||
|
||||
async function init_wasm(tid, memory, offset, notify_flags, stack_base) { |
||||
thread_id = tid; |
||||
buf_offset = offset; |
||||
|
||||
const result = await WebAssembly.instantiateStreaming(fetch('wasm/multi.wasm'), { |
||||
env: { 'memory': memory } |
||||
}); |
||||
|
||||
exports = result.instance.exports; |
||||
//console.log(tid, 'init');
|
||||
exports.set_sp(stack_base - thread_id * 4096); |
||||
|
||||
flags = notify_flags; |
||||
done(); |
||||
} |
||||
|
||||
function do_work(num, callback) { |
||||
//console.log(thread_id, 'work');
|
||||
exports.write_a_number(buf_offset, thread_id, thread_id * num); |
||||
done(); |
||||
} |
||||
|
||||
onmessage = (e) => { |
||||
if (e.data.type === 0) { |
||||
init_wasm(e.data.thread_id, e.data.memory, e.data.buffer_offset, e.data.flags, e.data.stack_base); |
||||
} else if (e.data.type === 1) { |
||||
do_work(e.data.num); |
||||
} |
||||
} |
||||
|
||||
|
Loading…
Reference in new issue