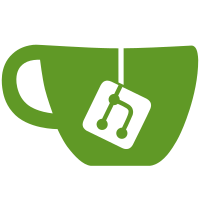
12 changed files with 177 additions and 127 deletions
@ -0,0 +1,35 @@
@@ -0,0 +1,35 @@
|
||||
const config = { |
||||
ws_url: `wss://${window.location.host}/ws/`, |
||||
ping_url: `https://${window.location.host}/api/ping`, |
||||
image_url: `https://${window.location.host}/images/`, |
||||
sync_timeout: 1000, |
||||
ws_reconnect_timeout: 2000, |
||||
brush_preview_timeout: 1000, |
||||
second_finger_timeout: 500, |
||||
buffer_first_touchmoves: 5, |
||||
debug_print: false, |
||||
zoom_delta: 0.05, |
||||
min_zoom_level: -250, |
||||
max_zoom_level: 100, |
||||
initial_offline_timeout: 1000, |
||||
default_color: 0x00, |
||||
default_width: 8, |
||||
bytes_per_instance: 4 * 2 + 4, // axy, stroke_id
|
||||
bytes_per_stroke: 2 * 3 + 2, // r, g, b, width
|
||||
initial_static_bytes: 4096 * 16, |
||||
initial_dynamic_bytes: 4096, |
||||
initial_wasm_bytes: 4096, |
||||
stroke_texture_size: 1024, // means no more than 1024^2 = 1M strokes in total (this is a LOT. HMH blackboard has like 80K)
|
||||
dynamic_stroke_texture_size: 128, // means no more than 128^2 = 16K dynamic strokes at once
|
||||
ui_texture_size: 16, |
||||
bvh_fullnode_depth: 5, |
||||
pattern_fadeout_min: 0.3, |
||||
pattern_fadeout_max: 0.75, |
||||
min_pressure: 50, |
||||
benchmark: { |
||||
zoom_level: -75, |
||||
offset: { x: 425, y: -1195 }, |
||||
frames: 500, |
||||
}, |
||||
}; |
||||
|
@ -0,0 +1,103 @@
@@ -0,0 +1,103 @@
|
||||
function undo(state, context, event, options) { |
||||
let need_draw = false; |
||||
|
||||
// Remove effect of latest own event, in a way that is recoverable
|
||||
|
||||
// Iterate back to front to find the _latest_ event
|
||||
for (let i = state.events.length - 1; i >=0; --i) { |
||||
const other_event = state.events[i]; |
||||
let skipped = false; |
||||
|
||||
// Users can only undo their own, undeleted (not already undone) events
|
||||
if (other_event.user_id === event.user_id && !other_event.deleted) { |
||||
// All "persistent" events (those that are pushed using SYN messages) should be handled here
|
||||
// "Transient" events are by design droppable, and should not be undone, nor saved in state.events at all
|
||||
switch (other_event.type) { |
||||
case EVENT.STROKE: { |
||||
other_event.deleted = true; |
||||
if (other_event.bvh_node && !options.skip_bvh) { |
||||
bvh_delete_stroke(state, other_event); |
||||
} |
||||
need_draw = true; |
||||
break; |
||||
} |
||||
|
||||
case EVENT.UNDO: { |
||||
// do not undo an undo, we are not Notepad
|
||||
skipped = true; |
||||
break; |
||||
} |
||||
|
||||
case EVENT.IMAGE: { |
||||
other_event.deleted = true; |
||||
const image = get_image(context, other_event.image_id); |
||||
if (image !== null) { |
||||
image.deleted = true; |
||||
} |
||||
need_draw = true; |
||||
break; |
||||
} |
||||
|
||||
case EVENT.IMAGE_MOVE: { |
||||
other_event.deleted = true; |
||||
const image = get_image(context, other_event.image_id); |
||||
if (image !== null) { |
||||
image.move_head -= 2; |
||||
image.at.x = image.move_history[image.move_head - 2]; |
||||
image.at.y = image.move_history[image.move_head - 1]; |
||||
need_draw = true; |
||||
} else { |
||||
console.warning('Undo image move for a non-existent image'); |
||||
} |
||||
break; |
||||
} |
||||
|
||||
case EVENT.IMAGE_SCALE: { |
||||
other_event.deleted = true; |
||||
const image = get_image(context, other_event.image_id); |
||||
if (image !== null) { |
||||
image.scale_head -= 4; |
||||
|
||||
// NEXT: merge move and scale. Otherwise we can't know
|
||||
// that there have been move events inbetween scale
|
||||
|
||||
image.at.x = image.scale_history[image.scale_head - 4]; |
||||
image.at.y = image.scale_history[image.scale_head - 3]; |
||||
image.width = image.scale_history[image.scale_head - 2]; |
||||
image.height = image.scale_history[image.scale_head - 1]; |
||||
need_draw = true; |
||||
} else { |
||||
console.warning('Undo image scale for a non-existent image'); |
||||
} |
||||
break; |
||||
} |
||||
|
||||
case EVENT.ERASER: { |
||||
other_event.deleted = true; |
||||
const stroke = state.events[other_event.stroke_id]; |
||||
stroke.deleted = false; |
||||
if (!options.skip_bvh) { |
||||
bvh_undelete_stroke(state, stroke); |
||||
} |
||||
need_draw = true; |
||||
break; |
||||
} |
||||
|
||||
default: { |
||||
console.error('cant undo event type', other_event.type); |
||||
break; |
||||
} |
||||
} |
||||
|
||||
if (!skipped) { |
||||
break; |
||||
} |
||||
} |
||||
} |
||||
|
||||
return need_draw; |
||||
} |
||||
|
||||
function redo() { |
||||
console.log('TODO'); |
||||
} |
Loading…
Reference in new issue